Introduction
In the realm of software development, one of the most ubiquitous and essential constructs is the console logging mechanism. It serves as a critical tool for developers, enabling them to monitor the flow of the program, debug issues, and understand the state of the application at any given point. Whether you’re a seasoned developer or a beginner stepping into the world of coding, the console log is likely one of the first things you learn to use and one you continue to rely on throughout your coding journey.
In the context of GridScript++, the console logging mechanism takes on even more significance. GridScript++ is a scripting language developed for use within the Grid Software Engine (SE). Given the diverse nature of tasks it is used for, ranging from simple calculations to complex operations, having a robust and flexible logging mechanism is absolutely necessary.
This article introduces and documents the console.log functionality that has been developed for GridScript++. We’ll explain the rationale behind the development of this construct, its utility in different execution contexts, and its ability to handle various data types. We’ll also provide examples of how to use it effectively in your GridScript++ code.
In GridScript++, console.log’s behavior depends on the execution context. If the thread executing the GridScript++ code is associated with a user interface, the console.log output will be displayed within a UI dialog box. On the other hand, if there’s no associated UI, the output will be directed to the terminal. This flexibility makes console.log a powerful tool for debugging and program flow monitoring in diverse usage scenarios.
Read on to learn more about the console.log construct in GridScript++, and how you can leverage it to enhance your GridScript++ programming experience.
How to Evaluate GridScript++
When you’re developing in GridScript++, you often need to quickly test pieces of code. To streamline this process, GridScript++ provides a useful utility command: ‘evalGPP’. This command allows developers to quickly execute GridScript++ code directly from the command line, without the need to create a new *.gpp file. This is especially useful for testing small snippets of code or for performing quick computations.
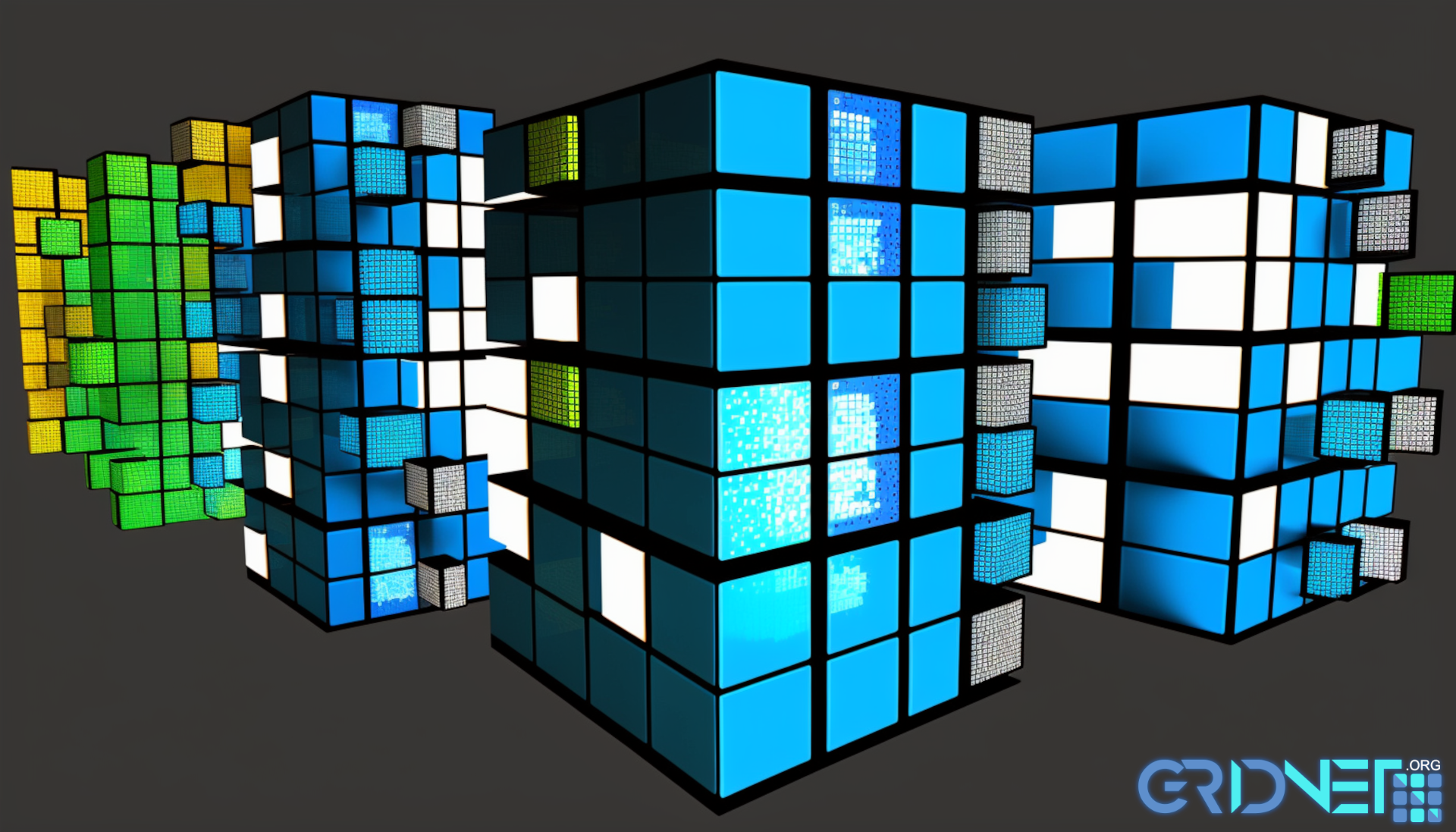
Fully object oriented ‘decentralized’ language still needs some of the basic constructs..
The syntax for the evalGPP command is as follows:
evalGPP [SOURCE]
Where `[SOURCE]` should be replaced with the GridScript++ source code you want to evaluate.
For example, if you wanted to quickly test a mathematical operation in GridScript++, you could run:
evalGPP "console.log(5 + 3);"
This would immediately evaluate the provided GridScript++ code (`console.log(5 + 3);`), logging the result (`8`) to the console.
The ‘evalGPP’ command can be used in two different environments: the Virtual Terminal interface available over SSH, and the Terminal UI dApp. In both cases, the command behaves the same way, providing a quick and easy way to test and debug your GridScript++ code.
In the Virtual Terminal over SSH, simply type the command directly into the terminal prompt. In the Terminal UI dApp, you can enter the command into the command input field and press Enter to execute it.
By leveraging the ‘evalGPP’ command, you can make your GridScript++ development process more efficient and agile, enabling rapid testing and iterative development.
Understanding console.log in GridScript++
The `console.log` function in GridScript++ is a versatile and powerful tool for developers to output information to the console or a UI dialog box, depending on the execution context. It helps developers debug their code, understand its behavior, and display useful information during the runtime of a GridScript++ program.
In this article, we will explore the functionality of `console.log` in GridScript++, its various supported data types, and how it adapts to different execution contexts.
Execution Contexts
`console.log` is designed to work seamlessly in different execution contexts, providing an optimal output method for each situation. When the thread executing GridScript++ code is associated with a user interface, `console.log` will display the content in a UI dialog box. In contrast, when executed in a non-UI context, such as a background task or a script executed over SSH, `console.log` will output the information to the terminal.
This adaptive behavior ensures that the output is always presented in the most appropriate and accessible manner for the current context, making it easier for developers to work with GridScript++ in various scenarios.
Supported Data Types
`console.log` in GridScript++ supports a wide range of data types to provide flexibility and convenience to developers. The following are the supported data types, along with sample code snippets demonstrating their usage:
1. String: Outputs a simple text message.
Strings are the most basic data type that developers often work with. They represent textual data and are used in almost every programming scenario. Developers might need to output strings to display messages, track the flow of their program, or debug by outputting variable values or function outputs as strings.
console.log("Hello, GridScript++!");
2. Number: Outputs a numeric value, including integers and floating-point numbers.
Numbers, both integers and floating-point, form the backbone of any calculations and logic in a script. Developers may need to output numbers to check calculated values, ensure that variables hold expected values, or display numerical results to the user.
console.log(42); console.log(3.14159);
3. Boolean: Outputs a boolean value, either `true` or `false`.
Boolean values represent a logical entity and can be either true or false. They’re often used in conditional statements and logic flows. Outputting boolean values can help developers verify the outcomes of logical operations, confirm the status of boolean flags, or trace the execution flow of conditional or loop statements.
console.log(true); console.log(false);
4. Array: Outputs an array, with each element logged recursively.
Arrays are used to store multiple values in a single variable. They are used extensively in data manipulation, sorting, searching, and many other operations. By outputting arrays, developers can check the content of the array at a particular moment, verify the outcome of an array operation, or monitor changes to the array over time.
var arr = [1, 2, 3, 4]; console.log(arr);
5. Object: Outputs a JavaScript object, with each key-value pair logged in a formatted manner.
JavaScript objects allow developers to group related data and functions. They represent real-world entities or complex data structures in the code. Developers can use console.log
to output the entire object and check the state of its properties, helping them to understand the state of the object at a specific point in time.
var car = {make: "Toyota", model: "Camry", year: 2023}; console.log(car);
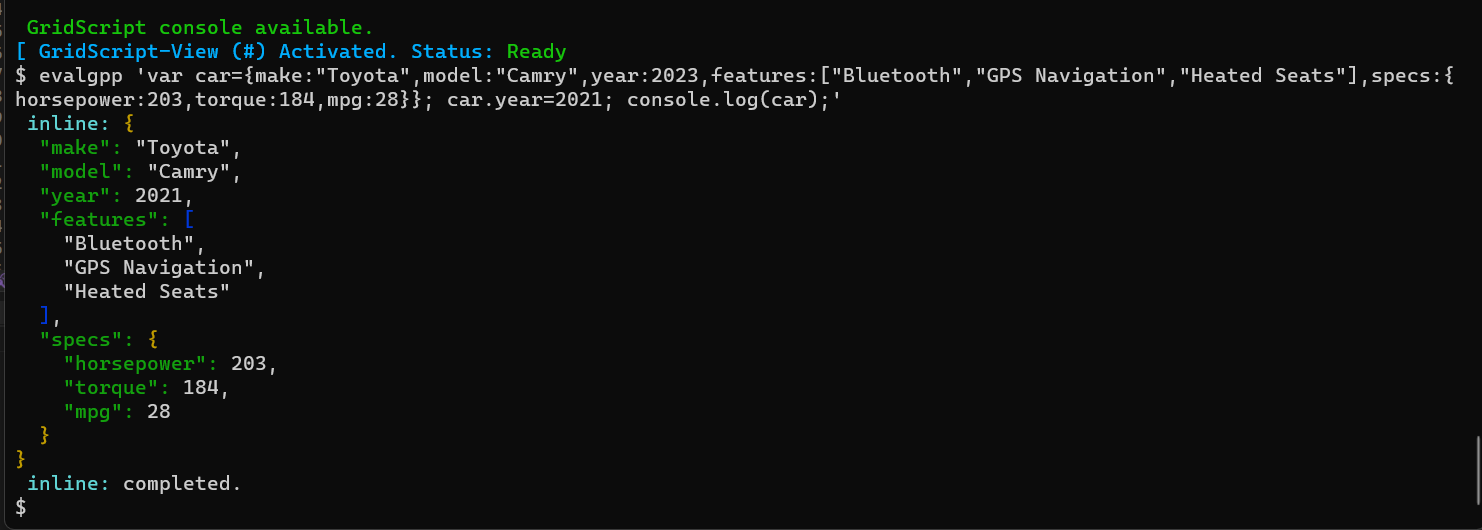
Pretty printed state of a GridScript++ object
6. ArrayBuffer and Buffer: Outputs ArrayBuffer and plain Buffer objects.
ArrayBuffers and Buffers represent a generic fixed-length raw binary data buffer. They are used when dealing with data streams, file operations, or any binary data manipulation. Developers can output these buffers to verify their content, size, or to debug buffer operations.
var buffer = new ArrayBuffer(8); console.log(buffer); var plainBuffer = new Buffer(8); console.log(plainBuffer);
By supporting these data types, `console.log` in GridScript++ offers a comprehensive solution for developers to output and examine different types of data during the execution of their programs.
In conclusion, `console.log` in GridScript++ is an essential tool for developers to effectively debug and display information during the runtime of their programs. Its adaptability to different execution contexts and support for a wide range of data types make it a valuable asset in the GridScript++ development process.
Automatic Data-Type Detection and Binary Data Handling
One of the powerful features of `console.log()` in GridScript++ is its ability to automatically detect the data type of the arguments passed to it. This takes away the tedious task of manually converting the data into a readable format before logging it. Now you can pass your variables directly to `console.log()`, and it will take care of the formatting for you.
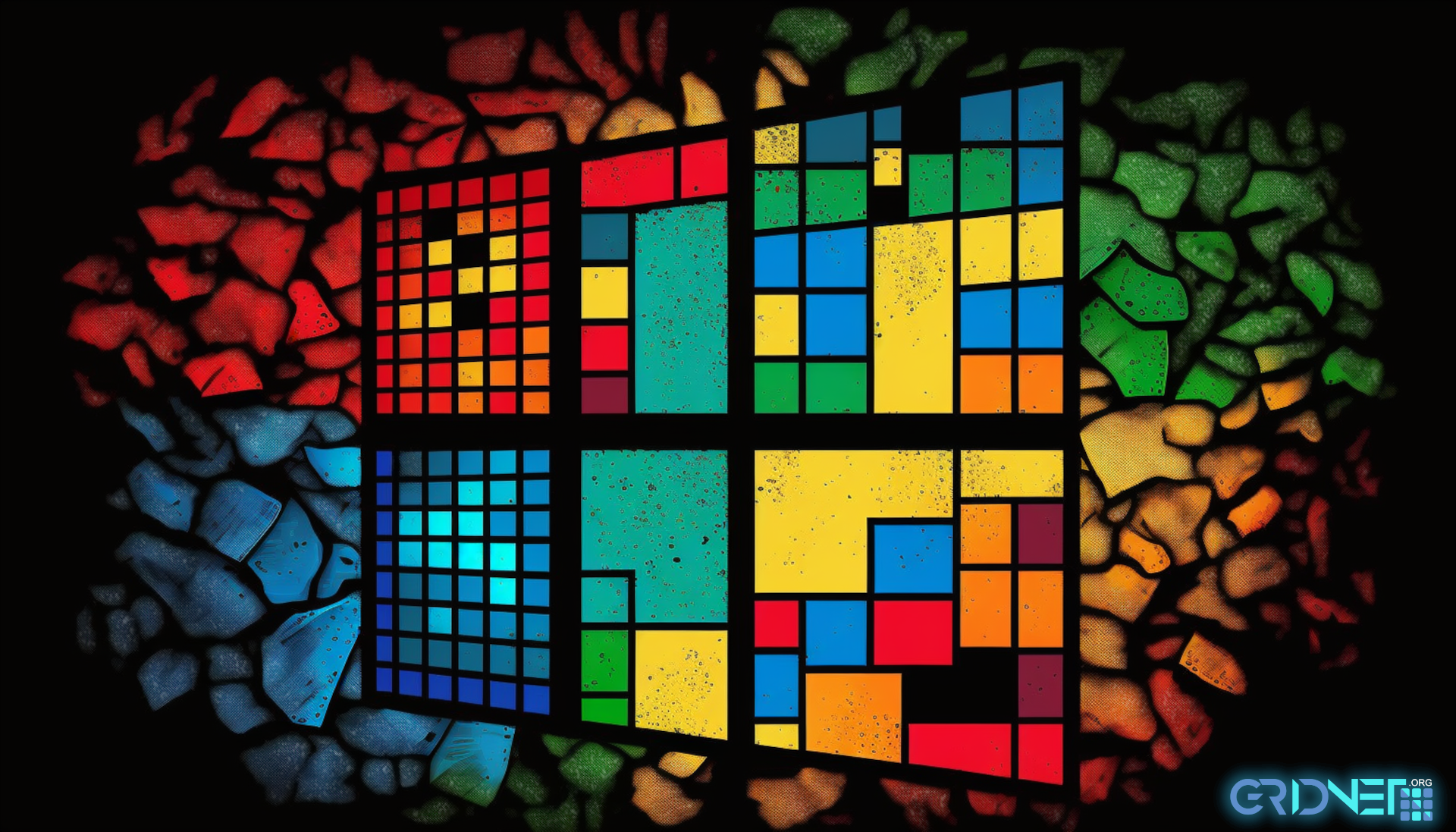
printf (“Characters: %c %c \n”, ‘z’, 80);… or?
This automatic detection is particularly beneficial when working with binary data and cryptographic constructs such as public and private keys. Cryptography is a core part of many modern software systems, and debugging these systems often involves inspecting binary data. However, binary data is not human-readable, and trying to print it out directly usually results in a stream of gibberish.
In GridScript++, when `console.log()` detects that it has been passed an `ArrayBuffer`, it doesn’t just dump the binary data directly to the console. Instead, it automatically encodes the data using base58-check encoding, transforming the binary data into a string of alphanumeric characters that can be read and compared more easily. This encoding is the same used across GridScript++ and GridScript 1.0 cryptographic constructs, making it easier to inspect and debug the cryptographic aspects of your code.
Indeed, here’s an example of how to use
console.log()
to print out a base58-check encoded public key:
var keyPair = GridCrypto.generateKeyPair(); console.log(keyPair.publicKey);
In this example, `keyPair.publicKey` is an `ArrayBuffer` containing the binary data of a public key. When we pass this to `console.log()`, it automatically encodes this `ArrayBuffer` into a base58-check encoded string before printing it to the console. This way, we can easily inspect the public key in a human-readable format.
This automatic handling of binary data is just one of the ways that `console.log()` in GridScript++ simplifies debugging and makes your code easier to understand. Whether you’re working with strings, numbers, objects, or binary data, `console.log()` has got you covered.